go-restful 剖析
k8s
的 APIServer
使用了go-restful
作为处理框架, 注册资源并处理HTTP
请求
在阅读APIServer
源码之前,需先理解go-restul
主要概念.
介绍(Intro)
来自github 的介绍: package for building REST-style Web Services using Go,go-restful
概念(Concepts)
Container
: 一组WebService
的集合, 目的:Containers for Webservices on different HTTP enpoints
.WebService
:Route
的集合; 为一组Route
定义统一的route path / 请求类型 / 响应类型
.Route
: 定义method/ULR path/调用函数/文档/参数/ curly route
; 支持正则及动态树。Filter
:Filters for intercepting the request --> response flow on service or ROute level
, 可以添加global /Webservice /Route
各自的Filter
初始化(Init Steps)
示例(Examples)
package main
import (
"github.com/emicklei/go-restful/v3"
"io"
"log"
"net/http"
)
func main() {
ws := new(restful.WebService)
ws.Route(ws.GET("/hello").To(hello))
restful.Add(ws)
log.Fatal(http.ListenAndServe(":8080", nil))
}
func hello(req *restful.Request, resp *restful.Response) {
io.WriteString(resp, "world")
}
多个container
示例
package main
import (
"github.com/emicklei/go-restful/v3"
"io"
"log"
"net/http"
)
func main() {
ws := new(restful.WebService)
ws.Route(ws.GET("/hello").To(hello1))
restful.Add(ws)
go func() {
log.Fatal(http.ListenAndServe(":8080", nil))
}()
container2 := restful.NewContainer()
ws2 := new(restful.WebService)
ws2.Route(ws2.GET("/hello").To(hello2))
container2.Add(ws2)
server := &http.Server{Addr:":8081", Handler: container2}
log.Fatal(server.ListenAndServe())
}
func hello1(req *restful.Request, resp *restful.Response) {
io.WriteString(resp, "default world")
}
func hello2(req *restful.Request, resp *restful.Response) {
io.WriteString(resp, "second world")
}
示例三: 包含多个filter
package main
import (
"github.com/emicklei/go-restful/v3"
"log"
"net/http"
"time"
)
type User struct {
Id, Name string
}
type UserList struct {
Users []User
}
// This example show how to create and use the three different Filters (Container,WebService and Route)
// When applied to the restful.DefaultContainer, we refer to them as a global filter.
//
// GET http://localhost:8080/users/42
// and see the logging per filter (try repeating this request)
func main() {
// install a global (=DefaultContainer) filter (processed before any webservice in the DefaultContainer)
restful.Filter(globalLogging)
restful.Add(NewUserService())
log.Print("start listening on localhost:8080")
log.Fatal(http.ListenAndServe(":8080", nil))
}
func NewUserService() *restful.WebService {
ws := new(restful.WebService)
ws.
Path("/users").
Consumes(restful.MIME_XML, restful.MIME_JSON).
Produces(restful.MIME_JSON, restful.MIME_XML)
// install a webservice filter (processed before any route)
ws.Filter(webserviceLogging).Filter(measureTime)
// install a counter filter
ws.Route(ws.GET("").Filter(NewCountFilter().routeCounter).To(getAllUsers))
// install 2 chained route filters (processed before calling findUser)
ws.Route(ws.GET("/{user-id}").Filter(routeLogging).Filter(NewCountFilter().routeCounter).To(findUser))
return ws
}
// Global Filter
func globalLogging(req *restful.Request, resp *restful.Response, chain *restful.FilterChain) {
log.Printf("[global-filter before (logger)] %s,%s\n", req.Request.Method, req.Request.URL)
chain.ProcessFilter(req, resp)
log.Printf("[global-filter after (logger)] %s,%s\n", req.Request.Method, req.Request.URL)
}
// WebService Filter
func webserviceLogging(req *restful.Request, resp *restful.Response, chain *restful.FilterChain) {
log.Printf("[webservice-filter before (logger)] %s,%s\n", req.Request.Method, req.Request.URL)
chain.ProcessFilter(req, resp)
log.Printf("[webservice-filter after (logger)] %s,%s\n", req.Request.Method, req.Request.URL)
}
// WebService (post-process) Filter (as a struct that defines a FilterFunction)
func measureTime(req *restful.Request, resp *restful.Response, chain *restful.FilterChain) {
now := time.Now()
chain.ProcessFilter(req, resp)
log.Printf("[webservice-filter (timer)] %v\n", time.Now().Sub(now))
}
// Route Filter (defines FilterFunction)
func routeLogging(req *restful.Request, resp *restful.Response, chain *restful.FilterChain) {
log.Printf("[route-filter before (logger)] %s,%s\n", req.Request.Method, req.Request.URL)
chain.ProcessFilter(req, resp)
log.Printf("[route-filter after (logger)] %s,%s\n", req.Request.Method, req.Request.URL)
}
// Route Filter (as a struct that defines a FilterFunction)
// CountFilter implements a FilterFunction for counting requests.
type CountFilter struct {
count int
counter chan int // for go-routine safe count increments
}
// NewCountFilter creates and initializes a new CountFilter.
func NewCountFilter() *CountFilter {
c := new(CountFilter)
c.counter = make(chan int)
go func() {
for {
c.count += <-c.counter
}
}()
return c
}
// routeCounter increments the count of the filter (through a channel)
func (c *CountFilter) routeCounter(req *restful.Request, resp *restful.Response, chain *restful.FilterChain) {
c.counter <- 1
log.Printf("[route-filter before (counter)] count:%d", c.count)
chain.ProcessFilter(req, resp)
log.Printf("[route-filter after (counter)] count:%d", c.count)
}
// GET http://localhost:8080/users
//
func getAllUsers(request *restful.Request, response *restful.Response) {
log.Print("getAllUsers")
response.WriteEntity(UserList{[]User{{"42", "Gandalf"}, {"3.14", "Pi"}}})
}
// GET http://localhost:8080/users/42
//
func findUser(request *restful.Request, response *restful.Response) {
log.Print("findUser")
response.WriteEntity(User{"42", "Gandalf"})
}
curl -i http://localhost:8080/users
curl -i http://localhost:8080/users/42
根据日志就可以看到具体流程了
「真诚赞赏,手留余香」
真诚赞赏,手留余香
使用微信扫描二维码完成支付
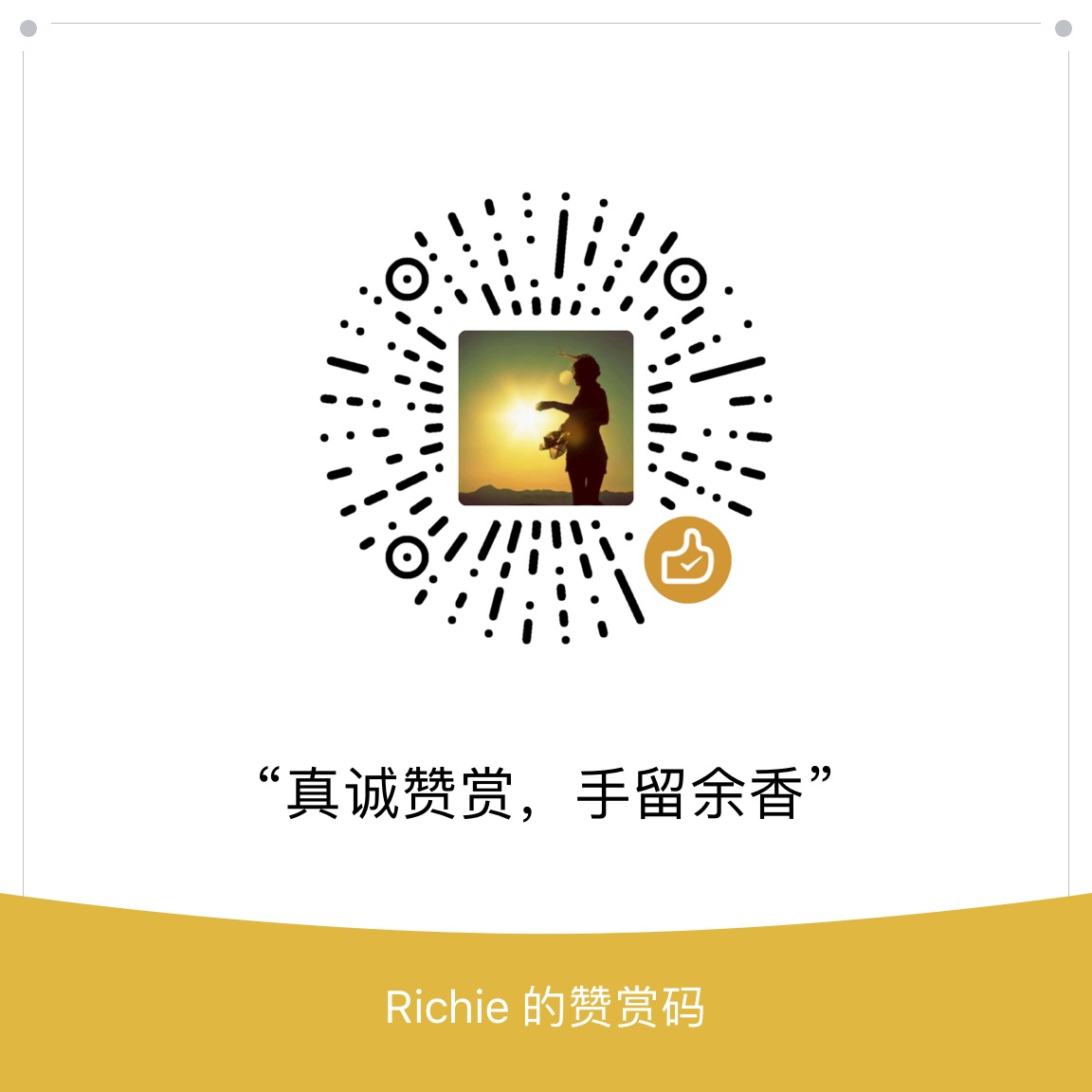